Android使用反射设置外部资源(SDK中使用居多)
- 作者: 我也不知道为什么
- 来源: 51数据库
- 2021-08-24
1、在开发SDK的过程中会发现通常是以jar和资源结合。而资源是提供给外部接入方使用的,在调用的时候为了防止编译的错误,要么使用反射,要么将资源放到jar工程当前,只是最后打包的时候不打资源包,将资源外放。这里提供下使用反射在sdk中调用外部资源的方法。
2、ReflectResourcesUtil类。调用前先调用init方法,当然如果你不想调用的话我也有备用的初始化方法,使用反射获取当前应用的Application来进行初始化。
import android.app.Application;
import android.content.Context;
import android.content.res.Resources;
import android.text.TextUtils;
import android.util.Log;
import java.lang.reflect.Method;
public class ReflectResourcesUtil {
private static final String TAG = "ReflectResourcesUtil";
private static String mPackageName;
private static Resources mResources;
/**
* 先调用初始化,然后再调用其他方法
*
* @param context
*/
public static void init(Context context) {
mPackageName = context.getApplicationContext().getPackageName();
mResources = context.getApplicationContext().getResources();
}
/**
* 防止没有调用初始化,使用备用初始化方法
*/
private static void retryInit() {
if (!TextUtils.isEmpty(mPackageName) || mResources != null) {
//已经初始化过,无需要再初始化
return;
}
Application application = getCurApplication();
if (application == null)
return;
init(application.getApplicationContext());
}
public static int getLayout(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "layout", mPackageName);
}
public static int getDrawable(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "drawable", mPackageName);
}
public static int getString(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "string", mPackageName);
}
public static int getId(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "id", mPackageName);
}
public static int getStyle(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "style", mPackageName);
}
public static int getArray(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "array", mPackageName);
}
public static int getColor(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "color", mPackageName);
}
public static int getDimen(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "dimen", mPackageName);
}
public static int getAnim(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "anim", mPackageName);
}
public static int getRaw(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "raw", mPackageName);
}
public static int getStyleable(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return -1;
}
return mResources.getIdentifier(resName, "styleable", mPackageName);
}
public static int[] getStyleableArray(String resName) {
retryInit();
if (mResources == null || TextUtils.isEmpty(mPackageName)) {
return null;
}
return getResourceIDsByName(resName, "styleable", mPackageName);
}
/**
* 利用反射,获取int数组格式的资源ID,例如styleable
*/
private static int[] getResourceIDsByName(String resName, String resType, String packageName) {
Class clsR = null;
int[] ids = null;
try {
clsR = Class.forName(packageName + ".R");
Class[] classes = clsR.getClasses();
Class desClass = null;
for (int i = 0; i < classes.length; i++) {
String[] temp = classes[i].getName().split("\\$");
if (temp.length >= 2) {
if (temp[1].equals(resType)) {
desClass = classes[i];
break;
}
}
}
if (desClass != null) {
ids = (int[]) desClass.getField(resName).get(resName);
}
} catch (Exception e) {
Log.e("ReflectResources", e.toString());
}
return ids;
}
/**
* 获取当前应用的Application
* 先使用ActivityThread里获取Application的方法,如果没有获取到,
* 再使用AppGlobals里面的获取Application的方法
*
* @return
*/
private static Application getCurApplication() {
Application application = null;
try {
Class atClass = Class.forName("android.app.ActivityThread");
Method currentApplicationMethod = atClass.getDeclaredMethod("currentApplication");
currentApplicationMethod.setAccessible(true);
application = (Application) currentApplicationMethod.invoke(null);
Log.d(TAG, "curApp class1:" + application);
} catch (Exception e) {
Log.d(TAG, "e:" + e.toString());
}
if (application != null)
return application;
try {
Class atClass = Class.forName("android.app.AppGlobals");
Method currentApplicationMethod = atClass.getDeclaredMethod("getInitialApplication");
currentApplicationMethod.setAccessible(true);
application = (Application) currentApplicationMethod.invoke(null);
Log.d(TAG, "curApp class2:" + application);
} catch (Exception e) {
Log.d(TAG, "e:" + e.toString());
}
return application;
}
}
推荐阅读
热点文章
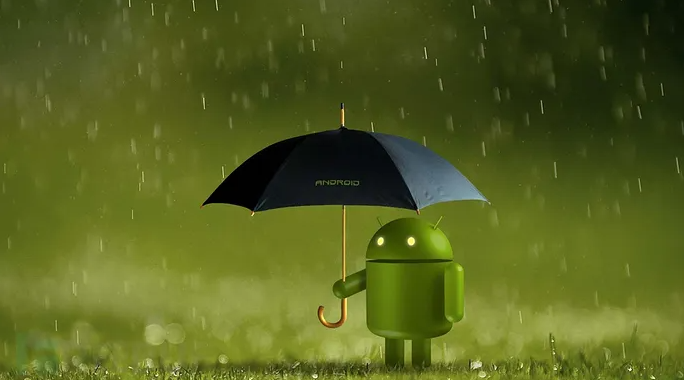
android中Bitmap用法(显示,保存,缩放,旋转)实例分析
12
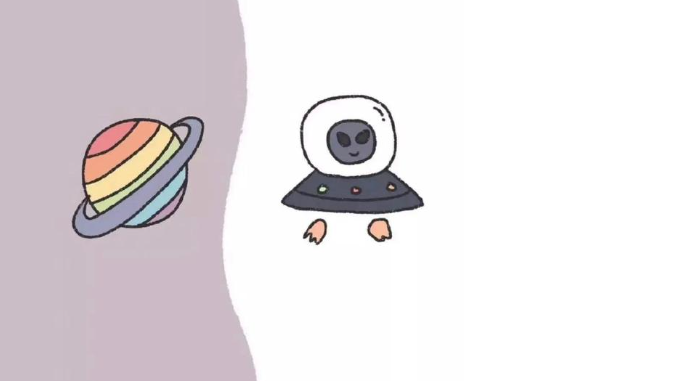
android 仿微信聊天气泡效果实现思路
1
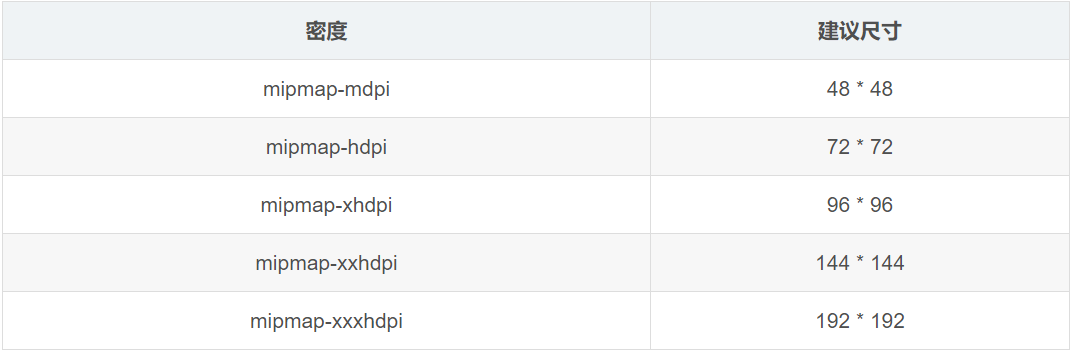
Android的尺度,drawable-xxxxxxx
2
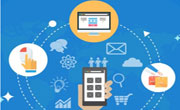
Codeforces Round #656 (Div. 3) (C、D题)
1
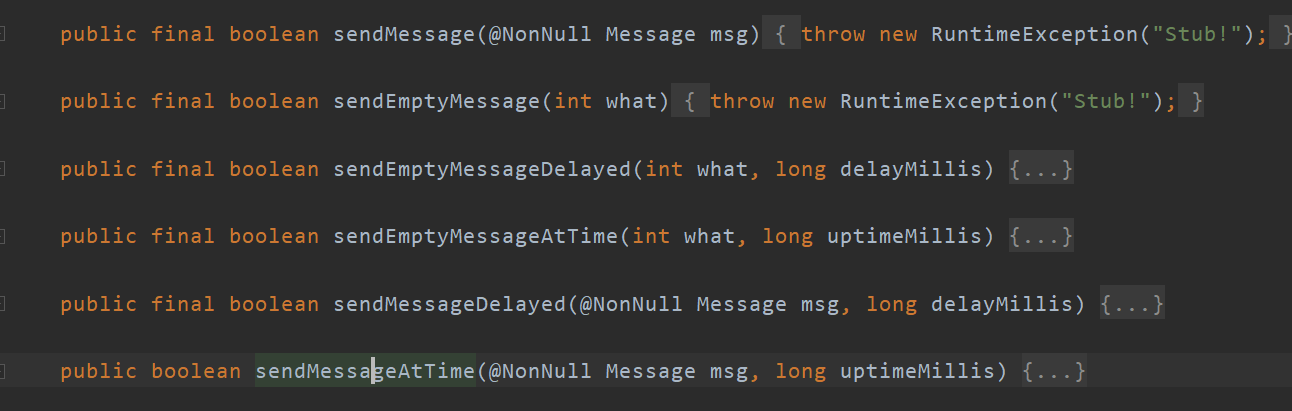
Android之handler异步消息处理机制解析
6
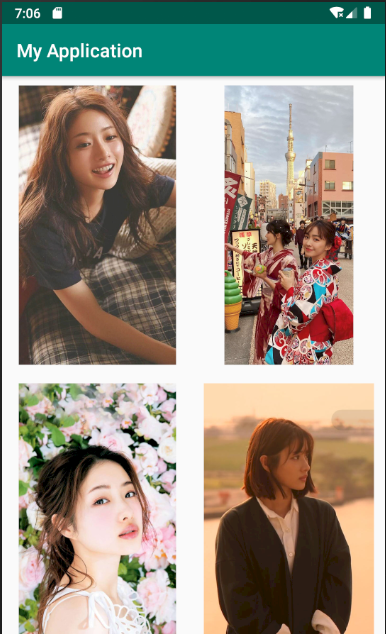
GridView中图片显示出现上下间距过大,左右图片显示类似瀑布流的问题
0
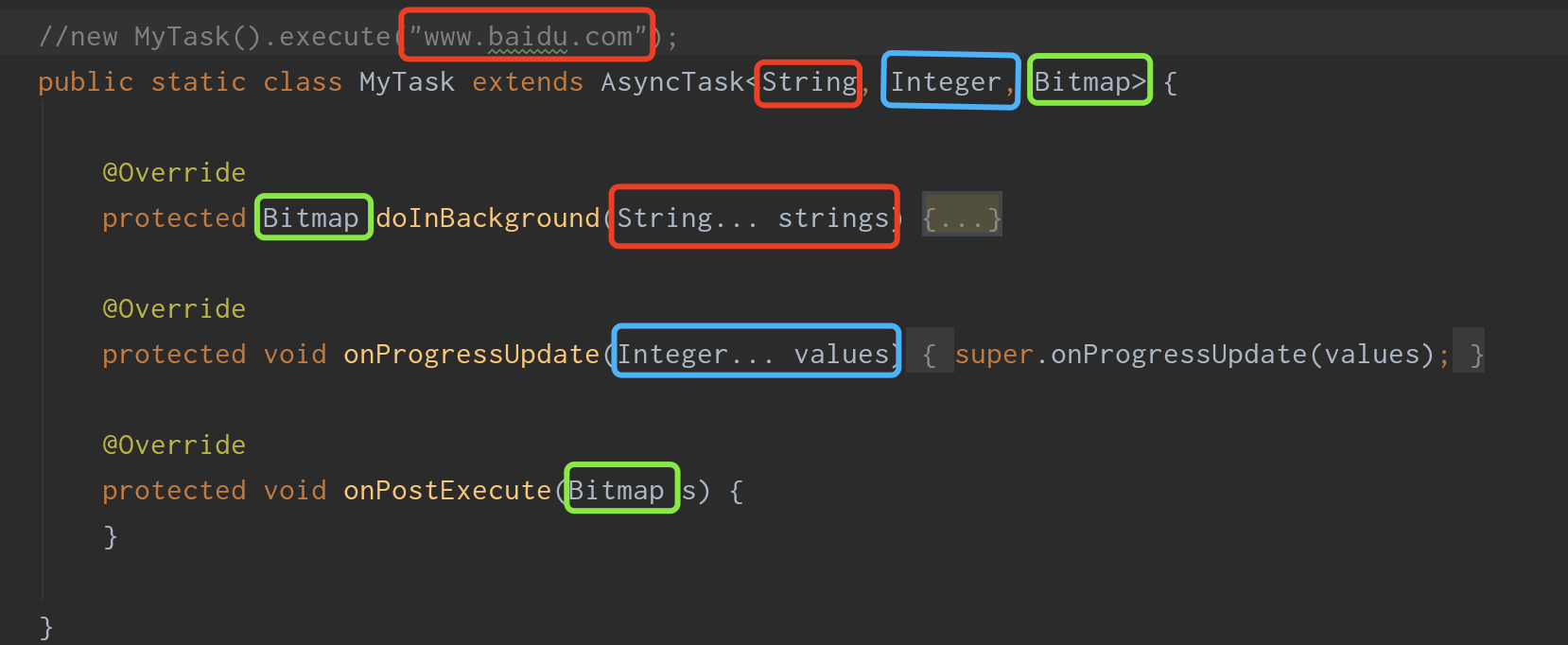
AsyncTask的简单使用
5
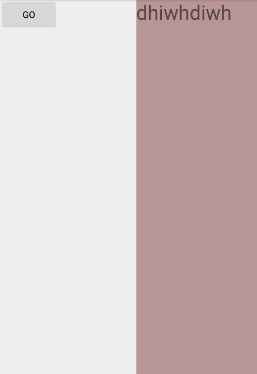
两个简单Fragment之间的通信(三种方式)
18
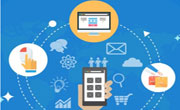
uboot修改设置boot参数命令
41
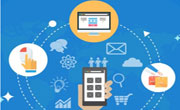
android中实现从相册中一次性获取多张图片与拍照,并将选中的图片显示出来
2