android 仿微信聊天气泡效果实现思路
- 作者: 80后的玩具
- 来源: 51数据库
- 2021-11-18
微信聊天窗口的信息效果类似iphone上的短信效果,以气泡的形式展现,在android上,实现这种效果主要用到listview和baseadapter,配合布局以及相关素材,就可以自己做出这个效果,素材可以下一个微信的apk,然后把后缀名改成zip,直接解压,就可以得到微信里面的所有素材了。首先看一下我实现的效果:
以下是工程目录结构:
接下来就是如何实现这个效果的代码:
main.xml,这个是主布局文件,显示listview和上下两部分内容。
复制代码 代码如下:
<?xml version="1.0" encoding="utf-8"?>
<relativelayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#f0f0e0" >
<relativelayout
android:id="@+id/rl_top"
android:layout_width="fill_parent"
android:layout_alignparenttop="true"
android:layout_height="wrap_content">
<textview
android:layout_width="fill_parent"
android:layout_height="44dp"
android:gravity="center"
android:textsize="18sp"
android:background="#486a9a"
android:textcolor="@android:color/white"
android:text="chat"/>
</relativelayout>
<relativelayout
android:id="@+id/rl_bottom"
android:layout_alignparentbottom="true"
android:layout_width="fill_parent"
android:background="#486a9a"
android:paddingtop="5dp"
android:layout_height="wrap_content">
<button
android:id="@+id/btn_send"
android:layout_width="70dp"
android:layout_height="50dp"
android:layout_alignparentright="true"
android:layout_centervertical="true"
android:layout_marginright="10dp"
android:text="send" />
<edittext
android:id="@+id/et_content"
android:layout_width="fill_parent"
android:layout_height="50dp"
android:layout_centervertical="true"
android:layout_marginleft="10dp"
android:layout_marginright="10dp"
android:layout_toleftof="@id/btn_send"
android:textsize="16sp"/>
</relativelayout>
<listview
android:id="@+id/listview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_above="@id/rl_bottom"
android:layout_below="@id/rl_top"
android:layout_marginleft="10dp"
android:layout_marginright="10dp"
android:layout_margintop="10dp"
android:cachecolorhint="#00000000"
android:divider="@null"
android:listselector="#00000000"
android:dividerheight="3dp"
android:scrollbars="none"/>
</relativelayout>
然后就是listview中两种类型item的布局文件,分别是接收信息的item效果和发送信息的item效果
chat_from_item.xml是接收信息的item布局:
复制代码 代码如下:
<?xml version="1.0" encoding="utf-8"?>
<linearlayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:orientation="vertical"
android:paddingbottom="5dp"
android:layout_height="wrap_content" >
<textview
android:id="@+id/tv_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:background="#bfbfbf"
android:paddingtop="2dp"
android:paddingbottom="2dp"
android:paddingleft="4dp"
android:paddingright="4dp"
android:textcolor="#ffffff"
android:textsize="12sp" />
<relativelayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_margintop="5dp" >
<imageview
android:id="@+id/iv_user_image"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_alignparentleft="true"
android:layout_alignparenttop="true"
android:background="@drawable/mypic"
android:focusable="false" />
<textview
android:id="@+id/tv_content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginleft="5dp"
android:layout_torightof="@+id/iv_user_image"
android:background="@drawable/chatfrom_bg"
android:gravity="left|center"
android:clickable="true"
android:focusable="true"
android:linespacingextra="2dp"
android:minheight="50dp"
android:textcolor="#ff000000"
android:textsize="14sp" />
</relativelayout>
</linearlayout>
chat_to_item.xml是发送信息item的布局:
复制代码 代码如下:
<?xml version="1.0" encoding="utf-8"?>
<linearlayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:orientation="vertical"
android:paddingbottom="5dp"
android:layout_height="wrap_content" >
<textview
android:id="@+id/tv_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#bfbfbf"
android:layout_gravity="center_horizontal"
android:paddingtop="2dp"
android:paddingbottom="2dp"
android:paddingleft="4dp"
android:paddingright="4dp"
android:textcolor="#ffffff"
android:textsize="12sp" />
<relativelayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_margintop="5dp" >
<imageview
android:id="@+id/iv_user_image"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_alignparentright="true"
android:layout_alignparenttop="true"
android:background="@drawable/mypic"
android:focusable="false" />
<textview
android:id="@+id/tv_content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginright="5dp"
android:layout_toleftof="@+id/iv_user_image"
android:background="@drawable/chatto_bg"
android:gravity="left|center"
android:clickable="true"
android:focusable="true"
android:linespacingextra="2dp"
android:textcolor="#ff000000"
android:textsize="14sp" />
</relativelayout>
</linearlayout>
布局完成后新建一个实体类chatentity.java:
复制代码 代码如下:
public class chatentity {
private int userimage;
private string content;
private string chattime;
private boolean iscomemsg;
public int getuserimage() {
return userimage;
}
public void setuserimage(int userimage) {
this.userimage = userimage;
}
public string getcontent() {
return content;
}
public void setcontent(string content) {
this.content = content;
}
public string getchattime() {
return chattime;
}
public void setchattime(string chattime) {
this.chattime = chattime;
}
public boolean iscomemsg() {
return iscomemsg;
}
public void setcomemsg(boolean iscomemsg) {
this.iscomemsg = iscomemsg;
}
}
最后就是主activity,这里面包括了自己写的baseadapter:
复制代码 代码如下:
public class chatdemoactivity extends activity {
private button sendbutton = null;
private edittext contentedittext = null;
private listview chatlistview = null;
private list<chatentity> chatlist = null;
private chatadapter chatadapter = null;
@override
public void oncreate(bundle savedinstancestate) {
super.oncreate(savedinstancestate);
requestwindowfeature(window.feature_no_title);
setcontentview(r.layout.main);
contentedittext = (edittext) this.findviewbyid(r.id.et_content);
sendbutton = (button) this.findviewbyid(r.id.btn_send);
chatlistview = (listview) this.findviewbyid(r.id.listview);
chatlist = new arraylist<chatentity>();
chatentity chatentity = null;
for (int i = 0; i < 2; i++) {
chatentity = new chatentity();
if (i % 2 == 0) {
chatentity.setcomemsg(false);
chatentity.setcontent("hello");
chatentity.setchattime("2012-09-20 15:12:32");
}else {
chatentity.setcomemsg(true);
chatentity.setcontent("hello,nice to meet you!");
chatentity.setchattime("2012-09-20 15:13:32");
}
chatlist.add(chatentity);
}
chatadapter = new chatadapter(this,chatlist);
chatlistview.setadapter(chatadapter);
sendbutton.setonclicklistener(new onclicklistener() {
@override
public void onclick(view v) {
if (!contentedittext.gettext().tostring().equals("")) {
//发送消息
send();
}else {
toast.maketext(chatdemoactivity.this, "content is empty", toast.length_short).show();
}
}
});
}
private void send(){
chatentity chatentity = new chatentity();
chatentity.setchattime("2012-09-20 15:16:34");
chatentity.setcontent(contentedittext.gettext().tostring());
chatentity.setcomemsg(false);
chatlist.add(chatentity);
chatadapter.notifydatasetchanged();
chatlistview.setselection(chatlist.size() - 1);
contentedittext.settext("");
}
private class chatadapter extends baseadapter{
private context context = null;
private list<chatentity> chatlist = null;
private layoutinflater inflater = null;
private int come_msg = 0;
private int to_msg = 1;
public chatadapter(context context,list<chatentity> chatlist){
this.context = context;
this.chatlist = chatlist;
inflater = layoutinflater.from(this.context);
}
@override
public int getcount() {
return chatlist.size();
}
@override
public object getitem(int position) {
return chatlist.get(position);
}
@override
public long getitemid(int position) {
return position;
}
@override
public int getitemviewtype(int position) {
// 区别两种view的类型,标注两个不同的变量来分别表示各自的类型
chatentity entity = chatlist.get(position);
if (entity.iscomemsg())
{
return come_msg;
}else{
return to_msg;
}
}
@override
public int getviewtypecount() {
// 这个方法默认返回1,如果希望listview的item都是一样的就返回1,我们这里有两种风格,返回2
return 2;
}
@override
public view getview(int position, view convertview, viewgroup parent) {
chatholder chatholder = null;
if (convertview == null) {
chatholder = new chatholder();
if (chatlist.get(position).iscomemsg()) {
convertview = inflater.inflate(r.layout.chat_from_item, null);
}else {
convertview = inflater.inflate(r.layout.chat_to_item, null);
}
chatholder.timetextview = (textview) convertview.findviewbyid(r.id.tv_time);
chatholder.contenttextview = (textview) convertview.findviewbyid(r.id.tv_content);
chatholder.userimageview = (imageview) convertview.findviewbyid(r.id.iv_user_image);
convertview.settag(chatholder);
}else {
chatholder = (chatholder)convertview.gettag();
}
chatholder.timetextview.settext(chatlist.get(position).getchattime());
chatholder.contenttextview.settext(chatlist.get(position).getcontent());
chatholder.userimageview.setimageresource(chatlist.get(position).getuserimage());
return convertview;
}
private class chatholder{
private textview timetextview;
private imageview userimageview;
private textview contenttextview;
}
}
}
对android&ios感兴趣的朋友可以加入我们的讨论qq群,在这里,我们只讨论干货:
ios群:220223507
android群:282552849
游戏开发论坛:http://jiushun8.com/forum.php?mod=viewthread&tid=4371&extra=page%3d1
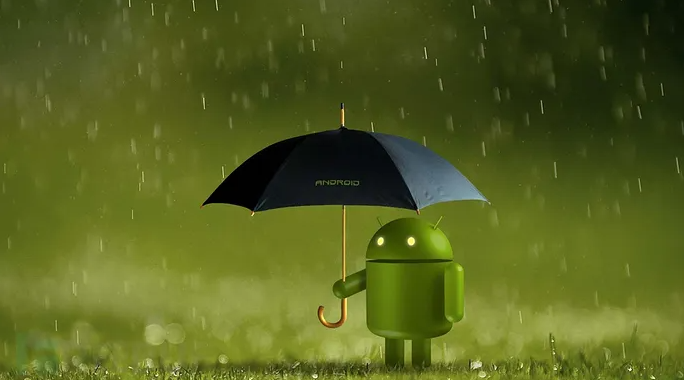
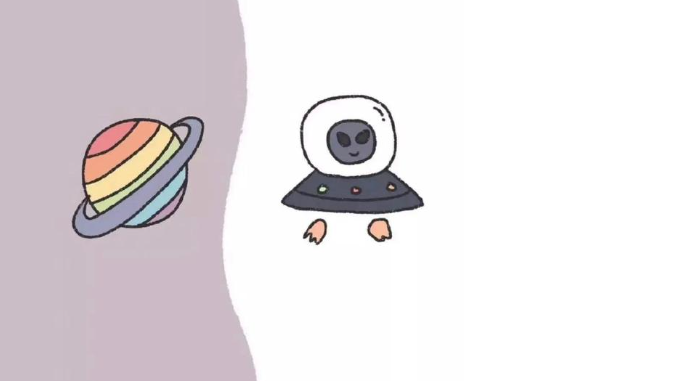
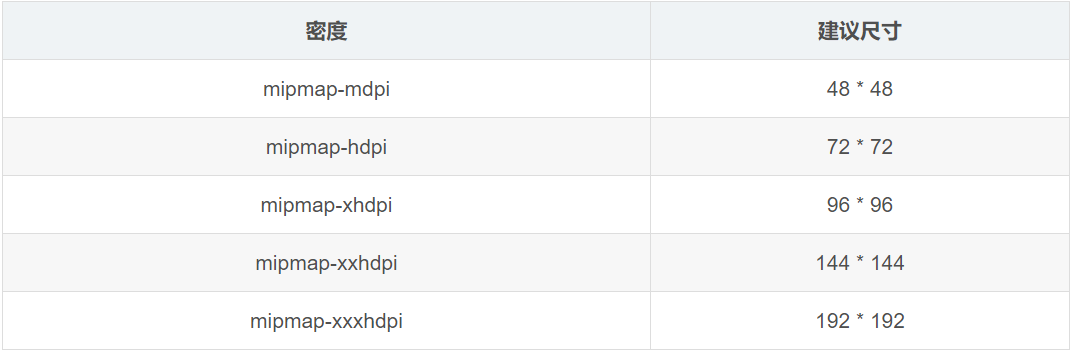
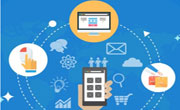
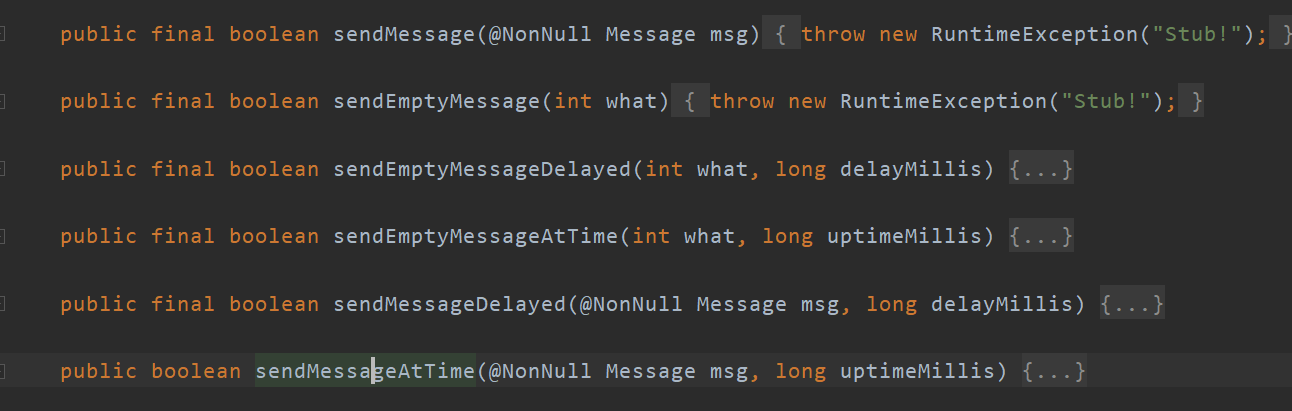
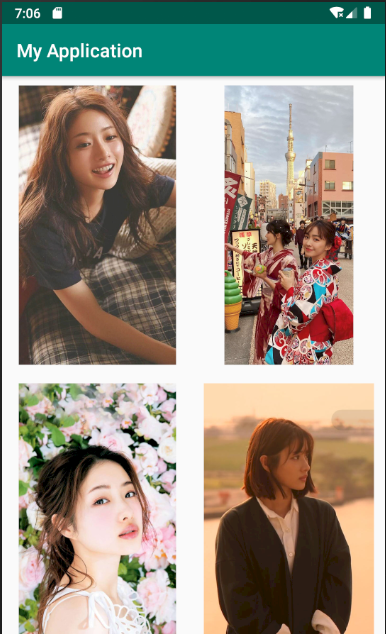
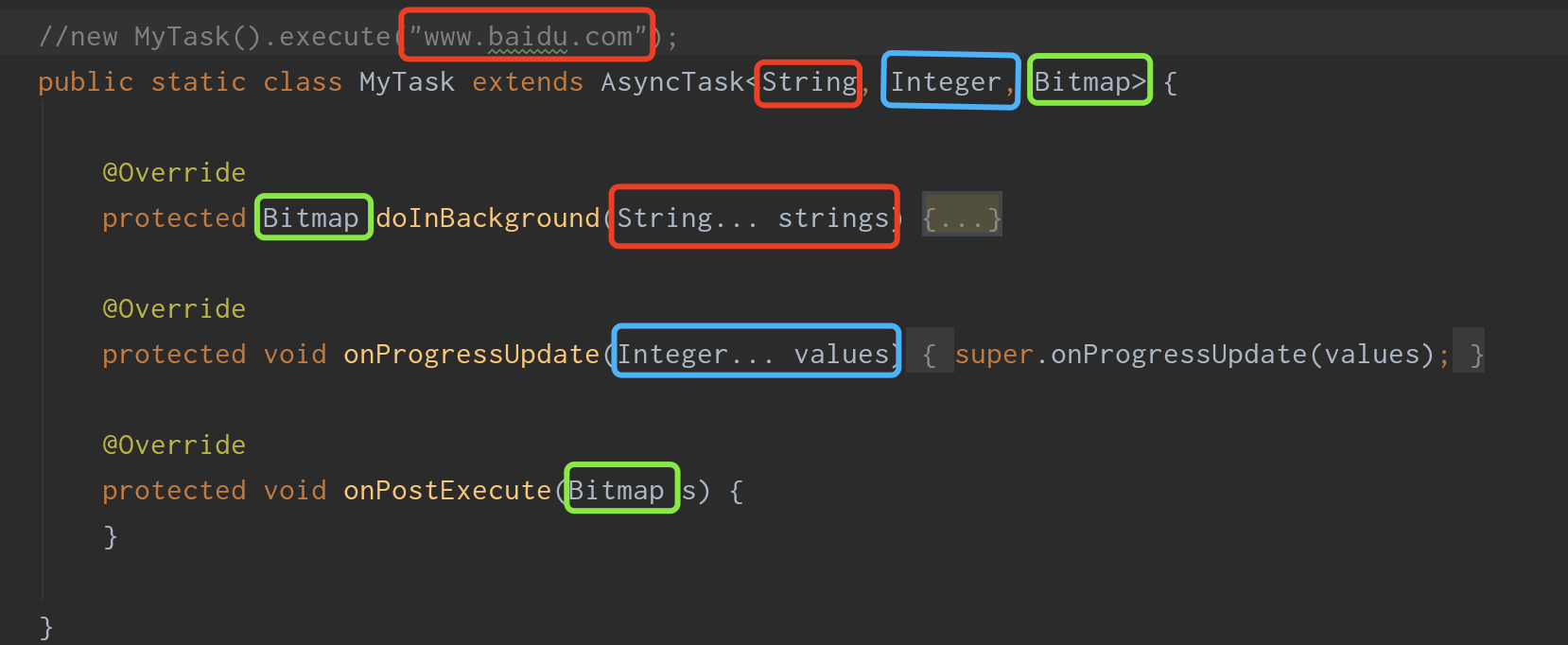
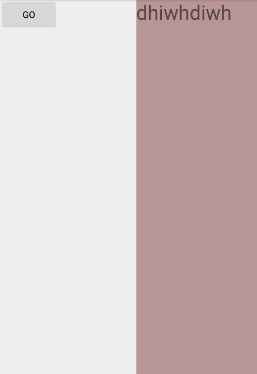
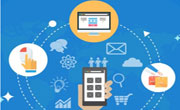
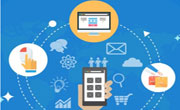