Android 自定义TextView,水平滚动
- 作者: 一个人一座城59217399
- 来源: 51数据库
- 2021-07-28
package com.my.androidtext.view; import android.content.Context; import android.graphics.Bitmap; import android.graphics.Canvas; import android.graphics.Paint; import android.os.Parcel; import android.os.Parcelable; import android.text.TextPaint; import android.util.AttributeSet; import android.view.View; import androidx.appcompat.widget.AppCompatTextView; public class HorizontalTextView extends AppCompatTextView { private float textLength = 0f; private float viewWidth = 0f; private float viewHeight = 0f; private float step = 0f; private float y = 0f; public boolean isStarting = false; private Paint paint = null; private Paint mBitPaint = null; private Bitmap mBitmap; private String text = ""; private boolean isInit = false; private boolean isCanScroll = true; private static final String POINT = "..."; private boolean textMarginRight = false; public HorizontalTextView(Context context) { super(context); initTextView(); } public HorizontalTextView(Context context, AttributeSet attrs) { super(context, attrs); initTextView(); } public HorizontalTextView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); initTextView(); } @Override public Parcelable onSaveInstanceState() { Parcelable superState = super.onSaveInstanceState(); SavedState ss = new SavedState(superState); ss.step = step; ss.isStarting = isStarting; return ss; } @Override public void onRestoreInstanceState(Parcelable state) { if (!(state instanceof SavedState)) { super.onRestoreInstanceState(state); return; } SavedState ss = (SavedState) state; super.onRestoreInstanceState(ss.getSuperState()); step = ss.step; isStarting = ss.isStarting; } public static class SavedState extends View.BaseSavedState { public boolean isStarting = false; public float step = 0.0f; SavedState(Parcelable superState) { super(superState); } @Override public void writeToParcel(Parcel out, int flags) { super.writeToParcel(out, flags); out.writeBooleanArray(new boolean[]{isStarting}); out.writeFloat(step); } public static final Creator<SavedState> CREATOR = new Creator<SavedState>() { public SavedState[] newArray(int size) { return new SavedState[size]; } @Override public SavedState createFromParcel(Parcel in) { return new SavedState(in); } }; private SavedState(Parcel in) { super(in); try { boolean[] b = null; in.readBooleanArray(b); if (b != null && b.length > 0) isStarting = b[0]; step = in.readFloat(); } catch (Exception e) { } } } public void setCanScroll(boolean canScroll) { isCanScroll = canScroll; } public void setTextMarginRight(boolean textMarginRight) { this.textMarginRight = textMarginRight; } private void startScroll() { isStarting = true; invalidate(); } private void stopScroll() { isStarting = false; invalidate(); } @Override public void onDraw(Canvas canvas) { if (!isInit) { initTextView(); } canvas.drawText(text, step, y, paint); if (!isStarting) { return; } step -= 1; if (-step > textLength) step = viewWidth; invalidate(); } @Override protected void onTextChanged(CharSequence text, int start, int lengthBefore, int lengthAfter) { super.onTextChanged(text, start, lengthBefore, lengthAfter); isInit = false; } private void initTextView() { paint = this.getPaint(); paint.setColor(getCurrentTextColor()); paint.setTextSize(getTextSize()); text = this.getText().toString().trim(); textLength = paint.measureText(text); step = 0; Paint.FontMetrics fontMetrics = paint.getFontMetrics(); ///文字一半的高度再减去基线到bottom的高度, float halfHeight = (fontMetrics.bottom - fontMetrics.top) / 2 - fontMetrics.bottom; y = getHeight() / 2 + (int) halfHeight; if (textLength > viewWidth) { if (isCanScroll) { startScroll(); } else { reInitTextWithPoint(); stopScroll(); } } else { if (textMarginRight) { step = viewWidth - textLength; } stopScroll(); } isInit = true; } private void reInitTextWithPoint() { TextPaint textPaint = new TextPaint(); textPaint.setTextSize(this.getTextSize()); while (textPaint.measureText(text) >= (viewWidth - textPaint.measureText(POINT))) { text = text.substring(0, text.length() - 1); } text += POINT; } @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { super.onMeasure(widthMeasureSpec, heightMeasureSpec); viewWidth = View.MeasureSpec.getSize(widthMeasureSpec); viewHeight = View.MeasureSpec.getSize(heightMeasureSpec); setMeasuredDimension((int) viewWidth, (int) viewHeight); } }
推荐阅读
热点文章
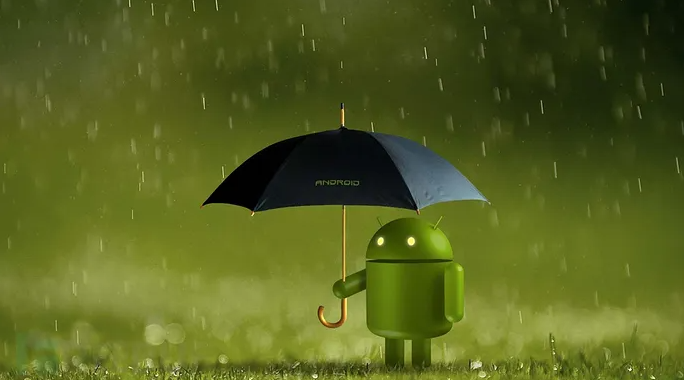
android中Bitmap用法(显示,保存,缩放,旋转)实例分析
12
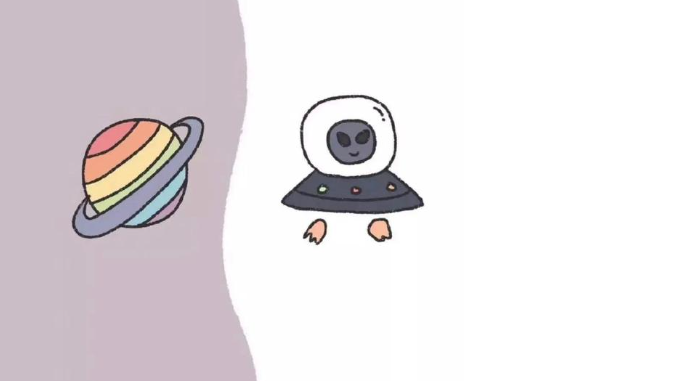
android 仿微信聊天气泡效果实现思路
1
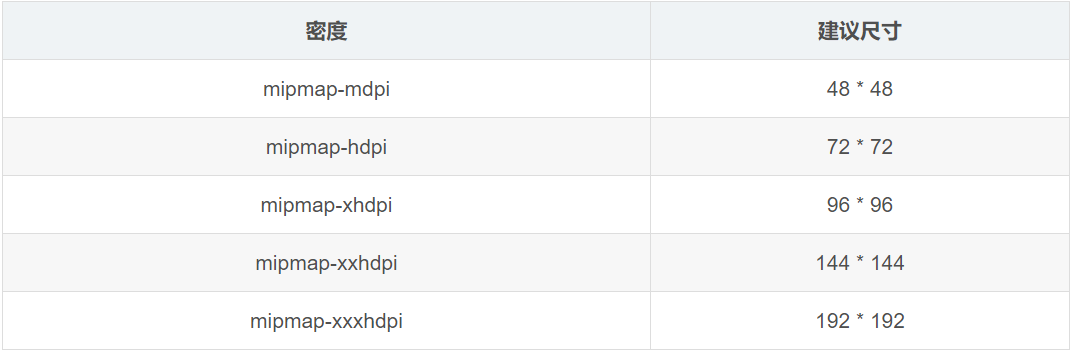
Android的尺度,drawable-xxxxxxx
2
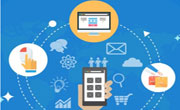
Codeforces Round #656 (Div. 3) (C、D题)
1
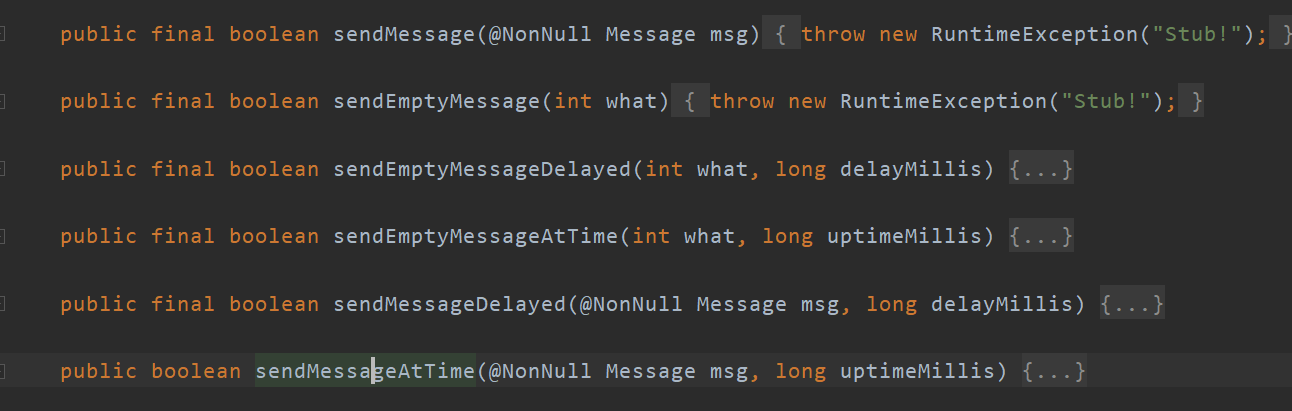
Android之handler异步消息处理机制解析
6
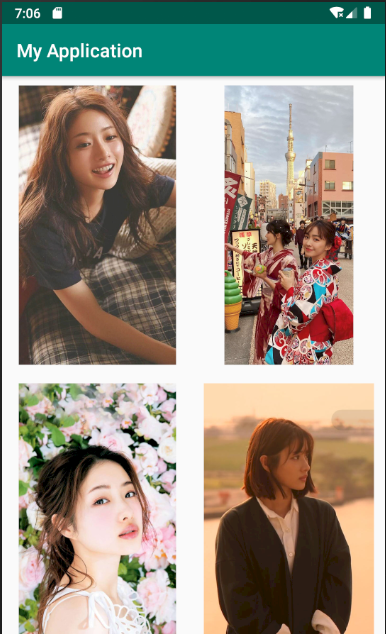
GridView中图片显示出现上下间距过大,左右图片显示类似瀑布流的问题
0
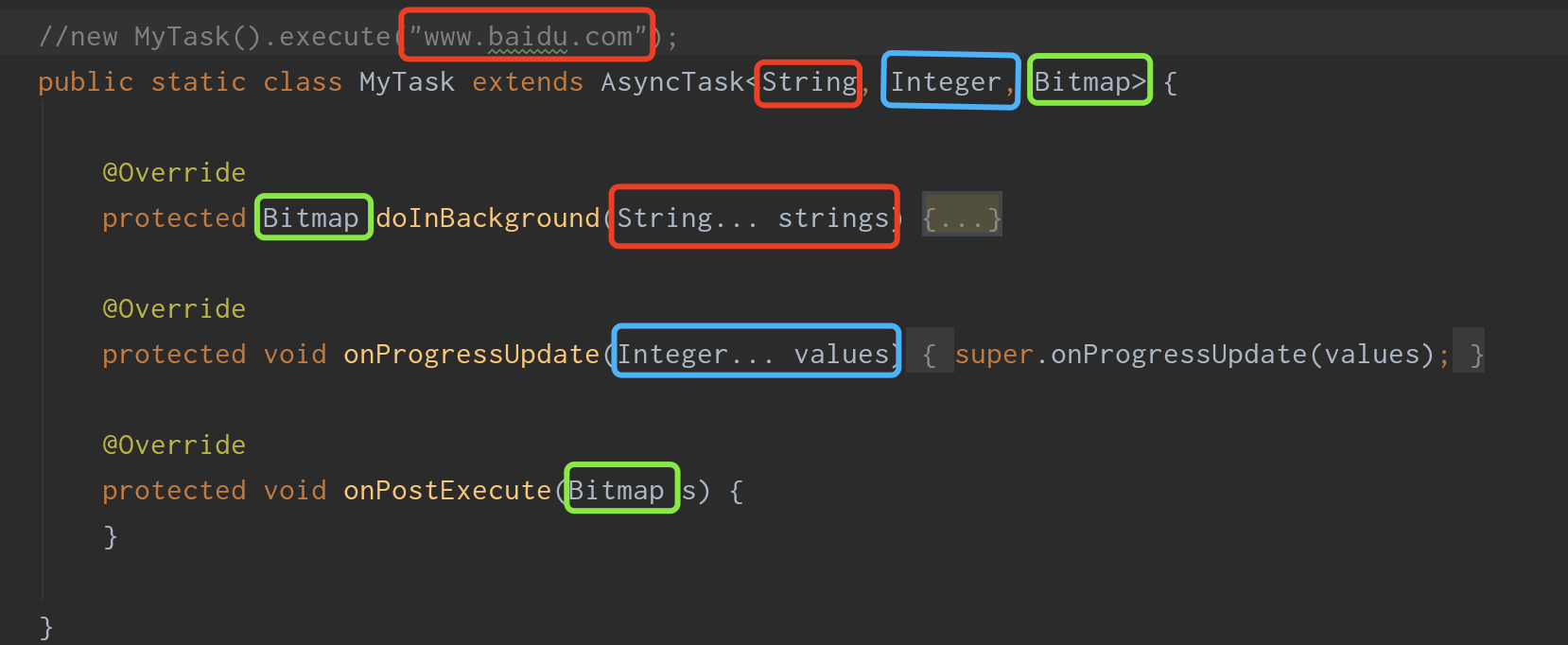
AsyncTask的简单使用
5
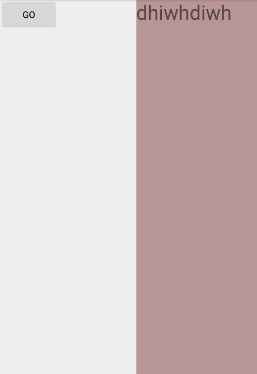
两个简单Fragment之间的通信(三种方式)
18
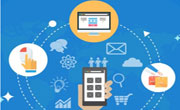
uboot修改设置boot参数命令
41
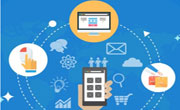
android中实现从相册中一次性获取多张图片与拍照,并将选中的图片显示出来
2