Android串口操作方法实例
- 作者: 率德布耀布耀德ljw
- 来源: 51数据库
- 2021-11-22
1.首先下载一个libserial_port.so,新建目录libs/armeabi,将so文件放到该目录下。
2.定义串口类,在类的构建函数中修改权限,打开设备,创建输入流和输出流,通过native接口访问串口打开关闭函数
复制代码 代码如下:
public class serialport {
/*do not remove or rename the field mfd: it is used by native method close();*/
public serialport(file device, int baudrate, int flags) throws securityexception, ioexception, invalidparameterexception{
//如果串口权限不够,改变权限
/* check access permission */
if (!device.canread() || !device.canwrite()) {
try {
/* missing read/write permission, trying to chmod the file */
process su;
su = runtime.getruntime().exec("/system/bin/su");
string cmd = "chmod 666 " + device.getabsolutepath() + "\n"
+ "exit\n";
su.getoutputstream().write(cmd.getbytes());
if ((su.waitfor() != 0) || !device.canread()
|| !device.canwrite()) {
throw new securityexception();
}
} catch (exception e) {
e.printstacktrace();
throw new securityexception();
}
}
mfd = open(device.getabsolutepath(), baudrate, flags);//打开串口
if (mfd == null) {
log.e(tag, "native open returns null");
throw new ioexception();
}
mfileinputstream = new fileinputstream(mfd);//串口输入流
mfileoutputstream = new fileoutputstream(mfd);//串口输出流
}
// getters and setters
public inputstream getinputstream() {
return mfileinputstream;
}
public outputstream getoutputstream() {
return mfileoutputstream;
}
// jni
private native static filedescriptor open(string path, int baudrate, int flags);//c文件中的串口open()函数
public native void close();//c文件中的串口close()函数
static {
system.loadlibrary("serial_port");//加载串口库
}
}
}
3.定义抽象类serverdata
复制代码 代码如下:
public abstract class serverdata {
protected serialport mserialport;
protected outputstream moutputstream;
private inputstream minputstream;
private readthread mreadthread;
private class readthread extends thread {
@override
//在线程中读取数据并处理数据
public void run() {
super.run();
byte[] buffer = new byte[128];
int size;
while(true) {
try {
if (minputstream == null) return;
size = minputstream.read(buffer);//读取数据
if (size > 0) {
ondatareceived(buffer, size);//处理数据
}
} catch (ioexception e) {
e.printstacktrace();
return;
}
}
}
}
4.实例化串口类,输出流和输入流,实例化读取线程并开始执行该线程
[code]
public serverdata(string path, int baudrate){
try {
mserialport = new serialport(new file(path), baudrate, 0);
moutputstream = mserialport.getoutputstream();
minputstream = mserialport.getinputstream();
/* create a receiving thread */
mreadthread = new readthread();
mreadthread.start();
} catch (securityexception e) {
} catch (ioexception e) {
} catch (invalidparameterexception e) {
}
}
protected abstract void ondatareceived(final byte[] buffer, final int size);
}
[/code]
5.然后再新建一个类,在新建的类中实现上面的抽象函数,并写一个函数返回读取到的数据。
复制代码 代码如下:
package view;
//导入r类,所在包不同,不能直接饮用,需要导入才可以使用
import android_serialport_api.sample.r;
/* etcview类,etc界面管理 */
public class serialview {
private activity context = null;
private serial metcserver = null;
/* etc界面构造函数 */
public serialview(activity context) {
this.context = context;
}
public void etcinitview() {
//这样才可以找到android_serialport_api.sample包下的id
textview mytext=(textview)context.findviewbyid(r.id.mytext);
metcserver = new serial("/dev/s3c2410_serial3", 9600);
}
public void etcrefresh() {
//返回串口线程读取的数据
byte[] buffer = metcserver.getdata();
string recstring=new string(buffer);//将byte[]的数组转换成字符串string
mytext.settext(recstring);//设置字符文本
buffer = null;
}
}
推荐阅读
热点文章
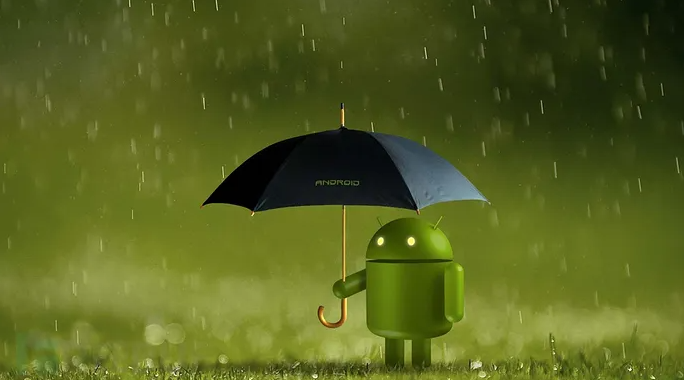
android中Bitmap用法(显示,保存,缩放,旋转)实例分析
12
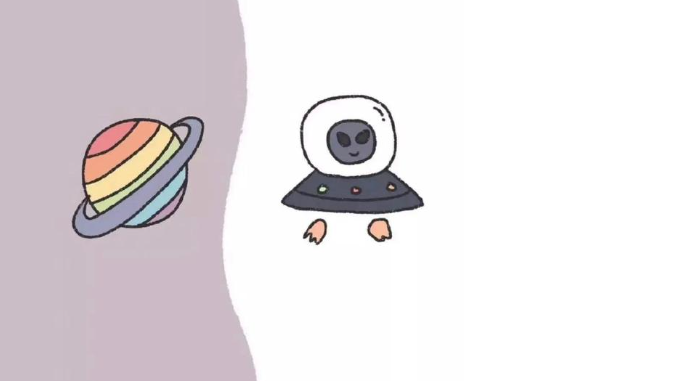
android 仿微信聊天气泡效果实现思路
1
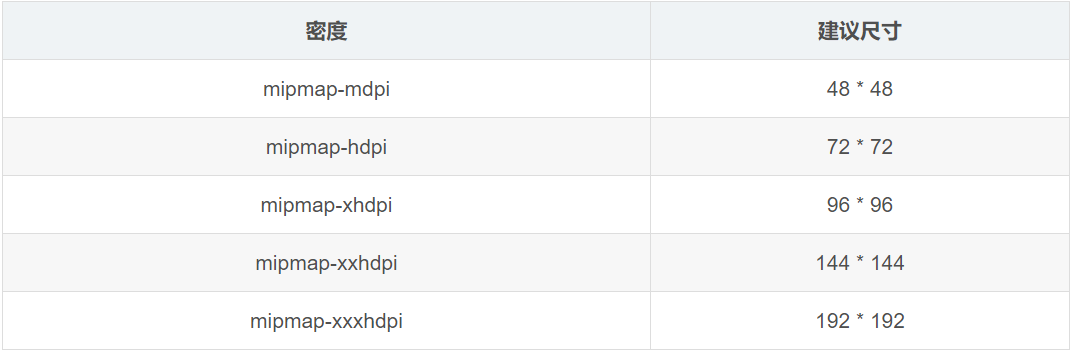
Android的尺度,drawable-xxxxxxx
2
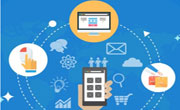
Codeforces Round #656 (Div. 3) (C、D题)
1
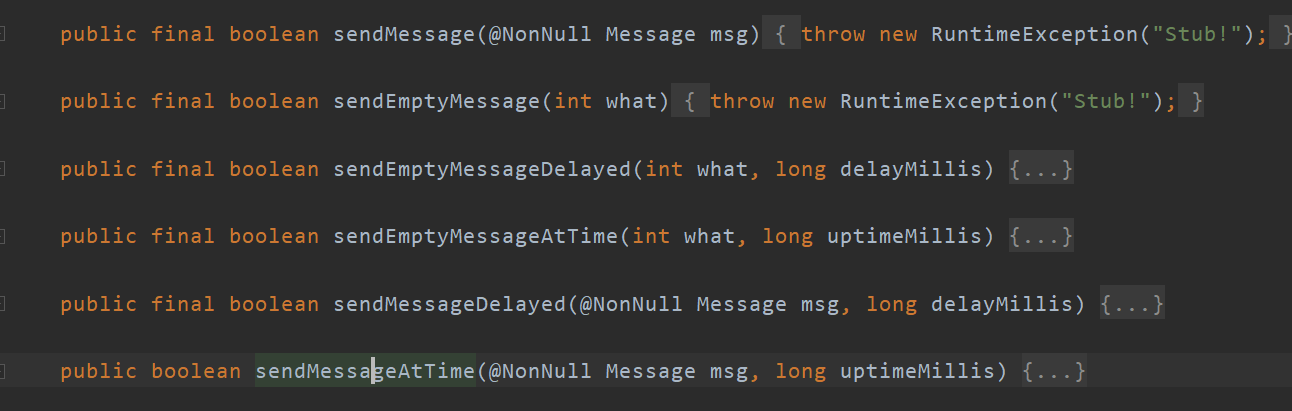
Android之handler异步消息处理机制解析
6
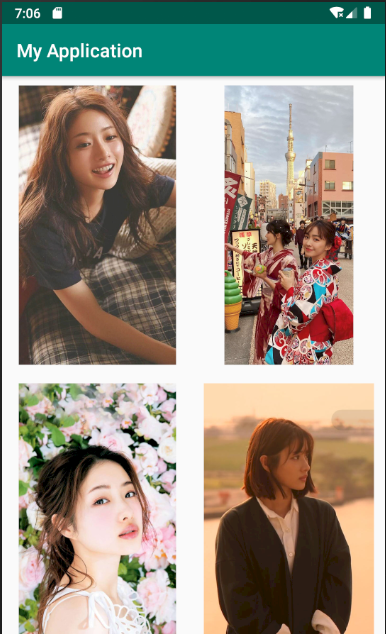
GridView中图片显示出现上下间距过大,左右图片显示类似瀑布流的问题
0
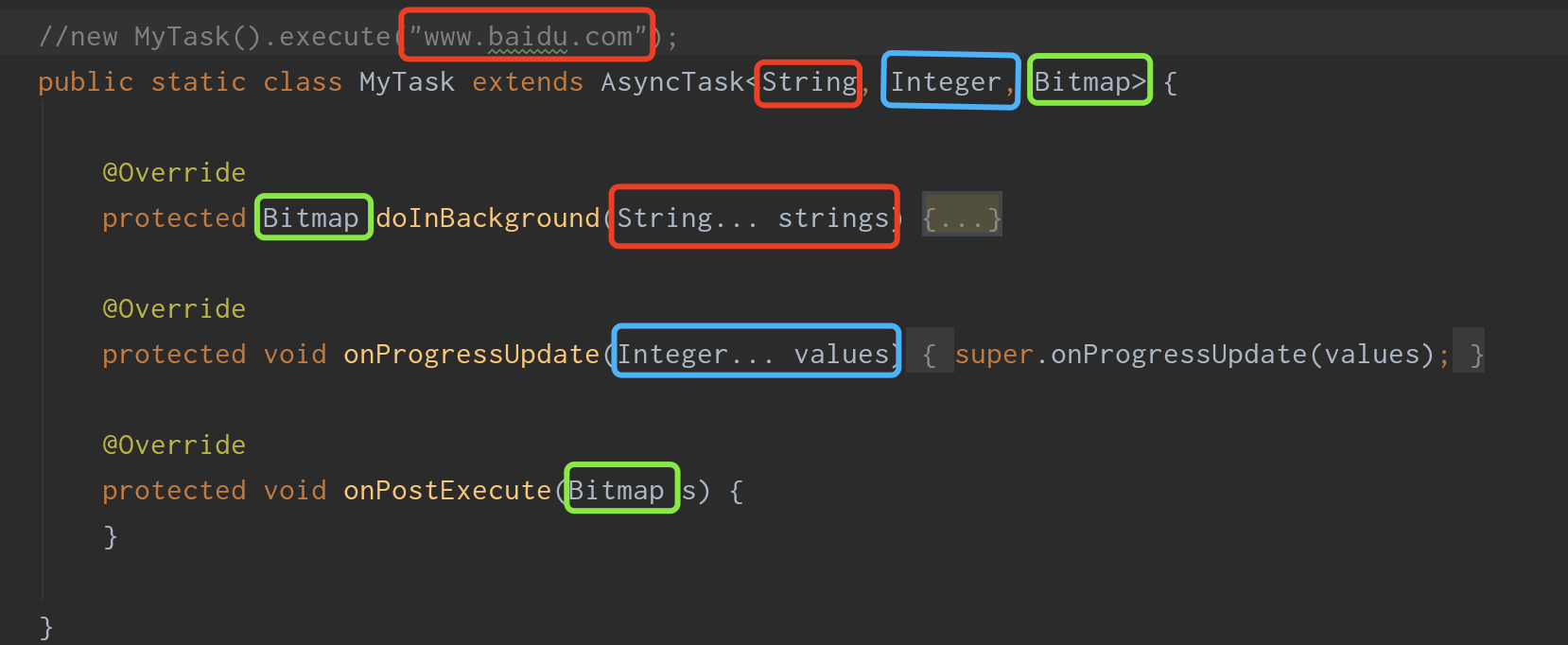
AsyncTask的简单使用
5
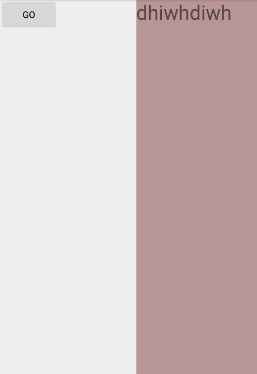
两个简单Fragment之间的通信(三种方式)
18
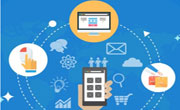
uboot修改设置boot参数命令
41
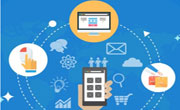
android中实现从相册中一次性获取多张图片与拍照,并将选中的图片显示出来
2